Introduction
APIs generally charge our applications with data. The client-side of the application consumes data from API which is normally in JSON format and is with specified endpoints. This information will look into the two ways, an application can consume an API. These ways are through ‘Fetch’ API which is a browser built-in API used to make the necessary HTTP requests and the Axios API which is defined as the promised-based HTTP client.
Shortly, we will cover these two methods of consuming the API in detail, distinguish them in the terms of their features and see how we could implement them.
Consuming an API with the Fetch() API
Fetch is JavaScript’s built-in API used to retrieve server responses or API endpoints. This APIs provides the fetch() method that retrieves the request’s responses. Unlike the Axios API, handling JSON data with fetch(), calls for a double process. The first step of fetch() API involves making an actual request by passing a ‘path’ or an ‘URL’ (from where the resource is to be fetched) to fetch() as a compulsory argument. The second step of this process is to call the json() method on the response that is returned as a promise from the request initially made. This converts the data response into JSON format before it is displayed on the browser.
Fetch possesses the advantage of being readily available in every modern browser like chrome, edge, and firefox and therefore doesn’t require installation, unlike Axios.
Passed parameters to the Fetch API
- Resource – The resource is the request’s path or the URL to be fetched.
- init object – the init object refers to the options that could initialize the fetch methods. The init object may include:
- Method: This specifies the HTTP request method used; ‘POST’, ‘PUT’, ‘GET’, ‘DELETE’.
- Headers: This entails any specific headers you would prefer added to the request. They are normally expressed in the form of an object or an object literal.
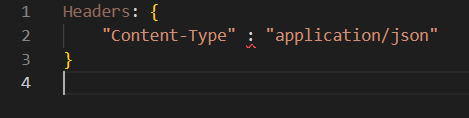
- Body: The body of data that would be added to the request.
- Mode: This is the mode to be added to the request either ‘cors’, or ‘no-cors’.
Fetch API basic syntax
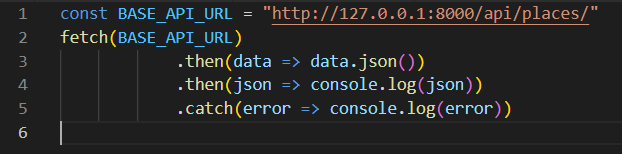
In the above code, the ‘BASE_API_URL’ variable is declared and the resource URL is passed to it. The ‘BASE_API_URL’ variable that points to the localhost URL for a REST API is passed to fetch from which the data is requested. A promise that contains a regular ‘HTTP’ response instead of the actual JSON (JavaScript Object Notation) format is returned. Therefore, using the .json() method on the returned data response convert the data into JSON format. In the case of any errors during data fetching from the API, they will be caught by the ‘. catch()’ method.
React Axios in Consuming data
The Axios is a promised-based JavaScript library that makes HTTP requests from node.js or XMLHttpRequests from the browser. It’s also a third-party package that needs to be installed.
Unlike in Fetch API where the data response needs to undergo a two-process conversion to JSON format, Axios automatically transforms the data response into JSON format.
Axios features
- The axios can easily intercept HTTP responses and HTTP requests.
- The axios are diversely supported by a range variety of browsers.
- It is provided built-in XSRF (Cross-site request forgery) protection.
- The canceling of requests as well as requests timeout is allowed by Axios.
- There is a built-in download and upload progress supported by Axios.
Axios Basic Syntax
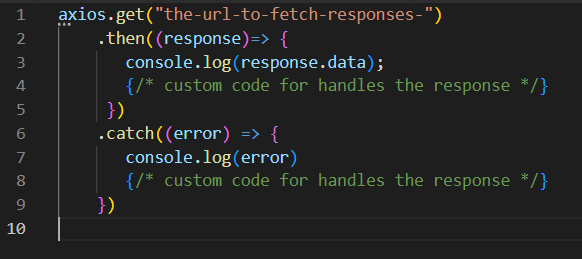
Making a ‘POST’ request with Axios
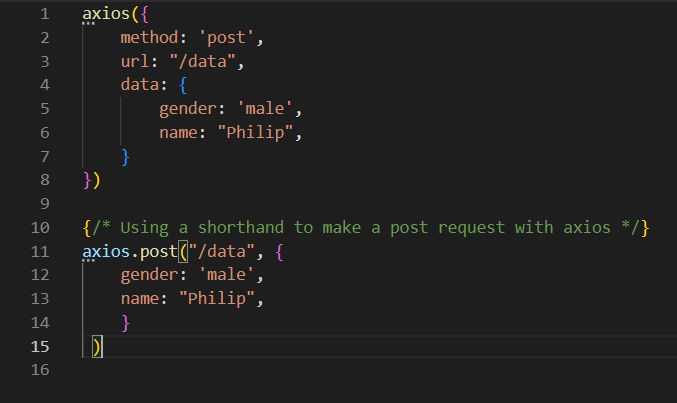
In the above code in that, the POST method is passed to the method’s object. The ‘/data’ is passed to the URL variable and refers to the specific endpoint to send the data (gender and name). The shorthand version of the code(axios.post({})) calls the post method directly to the axios and passes in the URL endpoint as the parameter.
Axios API vs Fetch API
It’s about time we compared these two APIs according to their distinct features and what exactly makes them suitable for use.
- It is easy to intercept HTTP requests. When changing these HTTP requests from any application to the server with the axios, there is no default way of intercepting requests using the fetch API.
- The axios allow making multiple HTTP requests while fetch() uses the ‘promise.all()’ method to provide the same feature. The following example explains this.
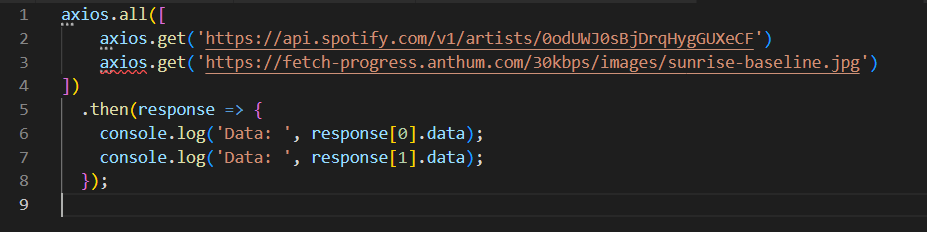
The above code fetches data from different URL sources and displays the responses to the console based on their indices; the first and the second response respectively.
- Another difference we have seen is browser compatibility where the axios are supported by a wide range of browsers whereas fetch() is limited for use in various of browsers like Chrome 42+, Firefox 39+, Edge 14+, and Safari 10.1+.
- Another difference is where Fetch is built-in while the axios is a third-party package that needs to be installed before it can be utilized.
- While this is not a feature for the two APIs, it is highly advisable to implement fetch API over Axios especially when developing smaller applications and implementing Axios for bigger applications for the sake of scalability.