What is State?
To make React Component Classes dynamic State is used. The state of a component is an object which holds information which may change the lifetime of the component.
State v Props
Once Props are set they can’t be changed so Props are immutable
- The state can hold data that may change over time so the state is observable
- Props can be used in function or class components
- The state is limited to class components
- The prop is set through the parent component
- Event handlers are used for updated state
Using State
- While the state is used, need the state of a component always exists — so need to set an initial state. By defining state in the constructor of the component class:
- class MyClass extends React.Component {
constructor(props){
super(props);
this.state = { attribute : “value” };
}
}
setState()
setState()
takes a single parameter and expects an object containing our set of values to be updated.- To re-render the page the method will update our state and then call the
render()
method. Below given a proper way to update our state,
· this.setState({attribute: “changed-value”});
- when we provide the initial state we should only ever be allowed to define our state explicitly in the constructor Lifecycle
What is the lifecycle?
Defining lifecycle means defining birth, growth & death. And React components follow this cycle as well: they’re created (mounted on the DOM), they experience growth (by updating) and they die (unmounted from the DOM). This is the component lifecycle!
There are different phases of the component lifecycle. each phase has its lifecycle methods.
The Lifecycle Methods
There are four parts of a component’s lifecycle
- Initialization
- Mounting
- Updating
- Unmounting
Initialization
In the initialization, the component will be setting its state & props. Initialization is usually done inside a constructor method
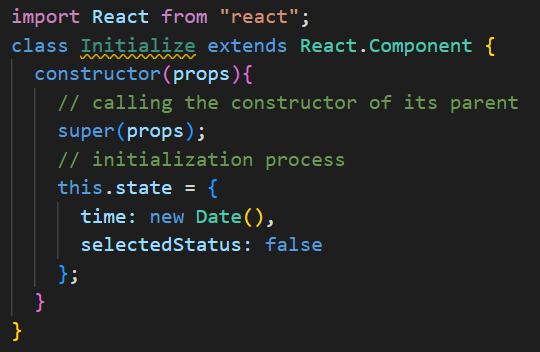
Mounting
Mounting is the phase in which component mounts on the DOM (i.e., is created and inserted into the DOM). The render method is called just before a component mounts on the DOM. After this method, the component gets mounted. componentWillMount()
and componentDidMount()
are the methods of this phase.
componentWillMount()
This method allows to execution of the React code synchronously when the component is mounted or gets loaded in the Document Object Model(DOM). This method is called during the mounting phase of the component Lifecycle.
This method is generally used to show a loader when the component is being loaded or when the data from the server is being fetched.
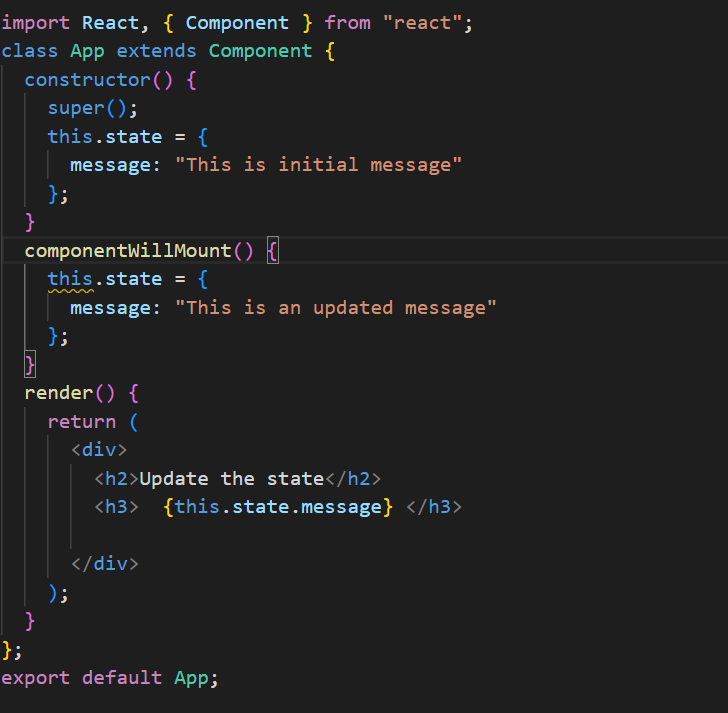
componentDidMount()
This method allows us to execute the code when the component is already placed in the DOM (Document Object Model). This method is called during the Mounting phase of the React component Life-cycle i.e after the component is rendered.
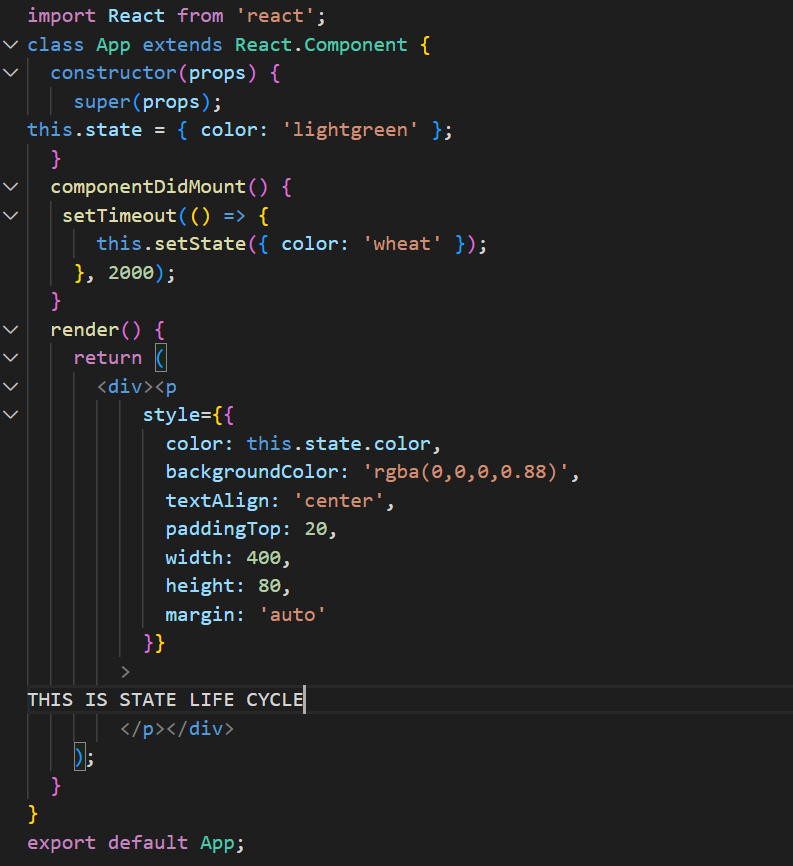
Updating
After completing the mounting phase where the component is created, we go to the update phase. Here the component’s state changes and thus, re-rendering takes place.
The data of the component may be stated or props will update in response to user events such as clicking, hovering, typing etc. These all responses result in the re-rendering of the component. Following three methods we use:
shouldComponentUpdate()
This method allows us to exit the complex react update life cycle to avoid calling it again and again on every re-render. The shouldComponentUpdate() only updates the component if the props passed to it change.
This method is used for optimizing the performance and to increase the responsiveness of the website/application but do not rely on it to prevent rendering as it may lead to bugs.
Syntax:
shouldComponentUpdate(nextProps, nextState)
componentWillUpdate()
This method is used during the updating phase of the React component lifecycle. The componentWillUpdate() function is generally called before the component is updated or when the state or props passed to the component changes. Don’t call setState() method in componentWillUpdate() function.
Note: This method is now deprecated in react.
componentDidUpdate()
This method allows us to execute the code when the component is updated. All the network requests that are to be made when the props are passed to the component changes are coded here.
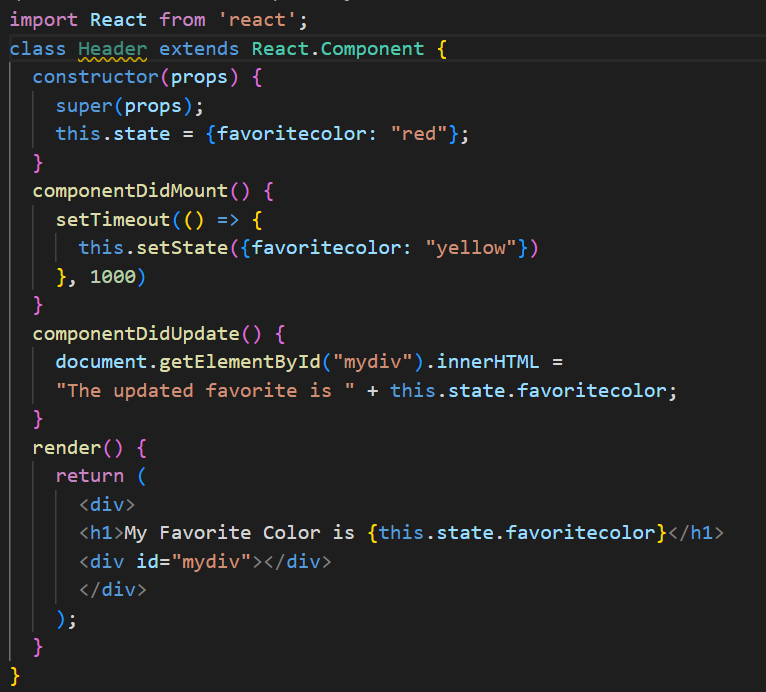
Unmounting
The last phase is unmounting. Where our component gets unmounted from the Document Object Model(DOM). The method we can use here is componentWillUnmount():
componentWillUnmount()
We call componentWillUnmount() method before the unmounting takes place. Before the removal of the component from the DOM, componentWillUnMount()
will execute. componentWillUnmount() method is the end of the component’s lifecycle!
Below is a flowchart neatly displaying all the lifecycle methods:
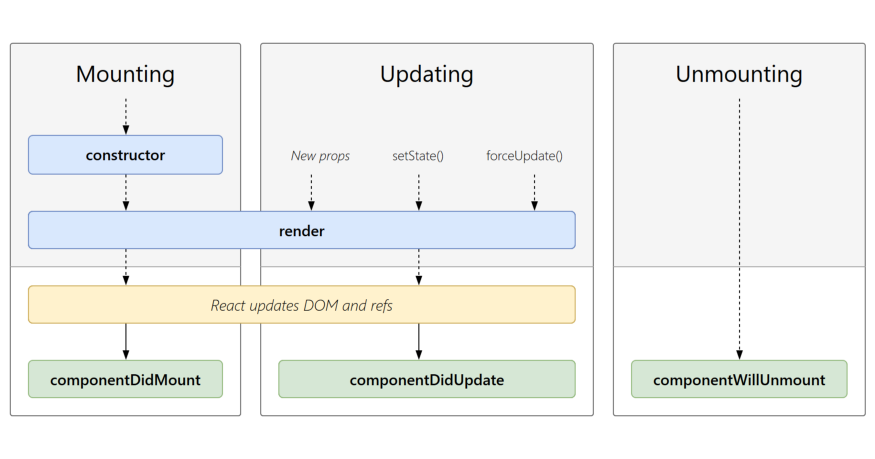
Conclusion
In this blog , firstly we see state and props and stateSet(). Then we moved on to the React Component Lifecycle, covering the phases (initialization, mounting, updating & unmounting) and the methods available to each.