Q. What are hooks?
Ans: Hooks are a new feature addition in react version 16.8, allowing you to use react features without writing a class. Hooks don’t work inside the classes. Ex. State of components. Hooks don’t contain any breaking changes and the hooks release is 100% backward-compatible
Motivation for hooks
Why did the react team always feel that there was a necessity for a react feature like hooks? This is the place where knowledge of react fundamentals and experience creating apps will help you better relate to the different reasons.
Reason 1:
Understand how this keyword works in JavaScript.
Always remember to bind event handlers in class components.
Class doesn’t minify very well and makes hot reloading very unreliable.
Reason 2:
There is no other particular way to reuse stateful component logic.
Higher order components and render props patterns do address this problem.
Makes the code harder to follow.
There is no need to share stateful logic in a better way.
Reason 3:
Create components for complex scenarios such as subscribing to the and events data fetching, and related code is not organized in one place
Ex. Data fetching- In componentDidMount and componentDidUpdate
Ex. Event listener- In componentDidMount and componentWillUnmount
We have stateful logic and that is the reason why we cannot break components into smaller ones.
Rules of Hooks:
- Only call hooks at the top level
In our logic, we don’t call hooks inside conditions, loops, or nested functions
- Only call hooks from react functions
Call hooks within react functional components and not just any regular javascript function.
- Hooks can not be conditional.
Types of Hooks
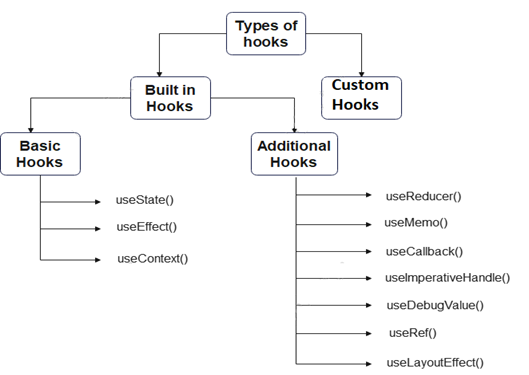
1.useState()
const [ state, setState ] = useState ( initialStateInput )
When first render, the returned state(state) is the same value passed as the first argument (initialStateInput). We want to set the new state, then the setState function is used to update the state. setState accepts the new state and enqueues a re-render of the component.
The useState hook returns a stateful value and a function to update it.
setState(newState)
2.useEffect() :
UseEffect is used to perform side effects on react functional components. Ex. Updating DOM (Document Object Model), fetching data from API endpoint, setting up subscriptions or timers. It is close replacement componentDidMount, componentWillUnmount, componentDidUpdate.
useEffect(()=>{
//runs on every render.
})
useEffect(()=>{
//runs only on the first render
},[]);
useEffect(()=>{
//runs on the first render
//and any time any dependency value changes
});
3. useContext() :
const value=useContext(MyContext)
The useContext accepts a context object (the value returned from React.createContext
) and returns the current context value for that context. The current context value is determined by the value
prop of the nearest <MyContext.Provider>
above the calling component in the tree.
The useContext provides a way to pass data through the component tree without having to pass props down manually at every level.
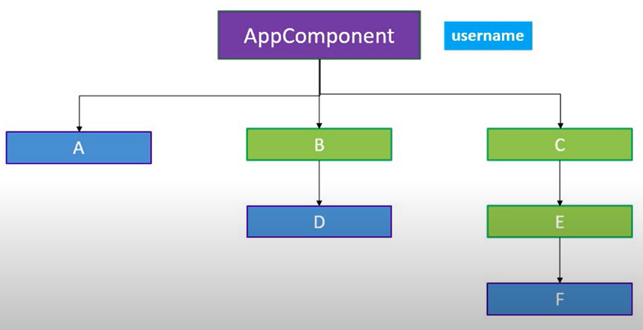
In the above picture, there is appComponent which is the root component. In that root component, several nested components are at different levels. At the foremost level, we have components A, B, and C. Next level B has D nested component and C has an E and F component.
Suppose we want to display the username in A, D, and F components that information is maintained as property in the app component. So, we have to pass props. It is easy to pass props from component C to F. IF the nested component increases at five or ten levels all the components in between would have to forward the prop this especially, becomes a problem for certain types of props such as language preference, UIP, and authenticated user which is pretty much required by many components in an application.
What would be nice is if we could directly send data to the required component without having manually drilled down props at every level of the component, this is where Context comes into the picture.
4. usReducer():
The useReducer is similar to useState(). If you have multiple pieces of state that rely on complex logic then useReducer() is the best way to use it.
useReducer(<reducer>, <initialState>)
It accepts two arguments. One is a reducer it contains custom state logic. Another one is initialState, it can be a value but generally contains an object. The useReducer returns the dispatch method and current state.
5. useRef():
The useRef can be used in DOM (Document Object Model) manipulation. While working with HTML we want to get value from the input box or change the color or focus a particular element, in some cases state and props are not working properly, in such cases we can use useRef().
const data =usRef();
6. useCallback():
The useCallback returns the memoized callback function. useCallback allows isolating resource-intensive functions so that it will not automatically run on every render. So that performance improves. useCallback run only when one of its dependencies update.
7. useMemo() :
The useMemo returns the memoized value. It runs only when one of its dependencies updates. It can improve performance.
8. useImpertiveHandle() :
useImpertiveHandle(ref , ceateHandle, [deps])
The
useImperativeHandle
customizes the instance value that is exposed to parent components when using ref
. As always, imperative code using the refs should be avoided in most cases.
9. useLayoutEffect() :
The useLayoutEffect is similar to the useEffect
, but useEffect fires synchronously after all DOM mutations. Use this to read the layout from the DOM(Document Object Model) and synchronously re-render. Updates scheduled inside the useLayoutEffect
will be flushed synchronously before the browser has a chance to paint.
10. useDebugValue() :
useDebugValue(value)
The useDebugValue hook
can be used to display a label for custom hooks in React extension (DevTools).
Custom Hooks :
Generally, hooks are reusable functions. All the above hooks are provided by the react library and these hooks functions in a way that they can be invoked within our components that can make you wonder. What is stopping us from creating these functions? Well, nothing is stopping us. We are even encouraged to build our hooks by extracting component logic into reusable functions, or in the simpler phrase, anyone can create their custom hook.
A custom hook is a javascript function whose name starts with the use keyword. A custom hook can also call other hooks if required.
When you have component logic that needs to be used by multiple components. So, we can
extract logic to a custom hook.
Why custom hooks?
Share logic- Alternative to HOCs and render props
WRAP UP:
Now, If we want to change our program’s game, start implementing all hooks and try to use them in different ways.
I hope, this blog is helpful for you to change the game of your program.
Thanks for Reading React developers.